Table of Contents
What is Rittinger’s Law?
Rittinger’s Law, proposed by the Rittinger in 1867, is a principle used to describe the energy consumption in size reduction processes such as crushing, grinding, and milling.
It states that the work required for size reduction of a solid material is directly proportional to the increase in surface area of the material. In simpler terms, the energy required to crush or grind a material is proportional to the new surface area created.
Mathematically, Rittinger’s Law can be expressed as:
\[ \frac{\dot{P}}{\dot{m}} = K_r \cdot \left( \frac{1}{D_{\text{final}}} – \frac{1}{D_{\text{initial}}} \right) \]
Where:
- \( \frac{\dot{P}}{\dot{m}} \) is the power per unit mass flow rate (\( \frac{P}{F} \)),
- \( K_r \) is Rittinger’s constant depending on the material and equipment used,
- \( D_{\text{final}} \) is the final particle size,
- \( D_{\text{initial}} \) is the initial particle size, and
- \( \dot{m} \) is the mass flow rate of the material being processed.
Note: Rittinger’s Law, often considered a hypothesis, the crushing efficiency remains constant i.e for a specific machine and feed material, efficiency does not vary with the sizes of the feed and product. Assuming equal sphericities (initial & final size) and constant mechanical efficiency, the constants involved can be combined into a single constant, denoted as \( K_r \).
Rittinger’s law have been shown to apply over limited ranges of particle size, provided that \( K_r \) is calculated experimentally by tests in a machine of the type to be used and with the material to be crushed.
Related: Kick’s Law Calculator
Related: Bond’s Law Calculator and Work Index
Rittinger’s Law calculator
The Rittinger‘s Law calculator helps user to calculate the energy required for crushing of a solid material based on Rittinger’s Law. It helps in predicting the energy needed to reduce the size of a given material from a specified initial size to a desired final size.
Related: Sphericity Calculator for different shapes
Significance of Rittinger’s Law
- It provides fundamental understanding of the relationship between energy consumption and particle size reduction. This is crucial in designing efficient crushing and grinding equipment in industries like mining, mineral processing, and chemical engineering.
- To understand the energy requirements for size reduction allows for optimization of processes to minimize energy consumption while achieving the desired particle size distribution.
- Efficient particle size reduction can lead to cost savings in terms of energy consumption and equipment maintenance.
Example Problem on Rittinger’s Law
A material is crushed in a Blake jaw crusher such that the average size of particle is reduced from 50 mm to 10 mm with the consumption of energy of 13.0 kW/(Kg/s). what would be consumption of energy needed to crush the same material of average size 75mm to an average of 25 mm, assuming Rittinger’s law applies to it?
Given:
Initial average particle diameter (\(D_1\)) = 50 mm \\
Final average particle diameter (\(D_2\)) = 10 mm \\
Energy consumed per unit mass flow rate (\(E_1\)) = 13.0 kW/(kg/s) \\
We know that Rittinger’s Law states:
\[ E_1 = K_R \left(\frac{1}{D_2} – \frac{1}{D_1}\right) \]
Solving for (\(K_R\)): \[ K_R = \frac{E_1}{\left(\frac{1}{D_2} – \frac{1}{D_1}\right)} \]
\[ K_R = \frac{13.0}{ \left(\frac{1}{10} – \frac{1}{50}\right)} = 162.5 \, \text{kW/kg·mm} \]
Second Scenario: Now, let’s find the energy consumption for the second scenario where the initial average particle diameter (\(D_1\)) is 75 mm, and the final average particle diameter (\(D_2\)) is 25 mm.
Using Rittinger’s Law again:
\[ E_2 = K_R \left(\frac{1}{D_2} – \frac{1}{D_1}\right) \]
Substituting the known values:
\[ E_2 = 162.5 \left(\frac{1}{25} – \frac{1}{75}\right) \]
Calculating: \[ E_2 = 4.33 \, \text{kW/(kg/s)} \]
Therefore, the energy consumption needed to crush the material from an average diameter of 75 mm to an average of 25 mm, assuming Rittinger’s law applies, is approximately 4.33 kW/(kg/s).
Python Code for Rittinger’s Law / Comminution Laws
This code calculates the power consumption for size reduction in a crushing process according to Rittinger’s Law. The initial and final particle sizes of the material being crushed, the mass flow rate of the material, and Rittinger’s constant are taken then plots the relationship between different values of Rittinger’s constant and the corresponding power consumption in kilowatts (kW) for the given process parameters.
Note: This Python code solves the specified problem. Users can copy the code and run it in a suitable Python environment. By adjusting the input parameters, users can observe how the output changes accordingly.
import matplotlib.pyplot as plt
def calculate_power_consumption(K_r, D_final_mm, D_initial_mm, mass_flow_rate_ton_hr):
"""
Calculate the power consumption for size reduction using Rittinger's Law.
Args:
- K_r: Rittinger's constant (in kW*hr*m/ton).
- D_final_mm: Final diameter of the material after crushing (in millimeters).
- D_initial_mm: Initial diameter of the feed material (in millimeters).
- mass_flow_rate_ton_hr: Mass flow rate of the material (in tons per hour).
Returns:
- Power consumption for size reduction (in kilowatts).
"""
D_final = D_final_mm / 1000 # Convert millimeters to meters
D_initial = D_initial_mm / 1000 # Convert millimeters to meters
mass_flow_rate_kg_s = mass_flow_rate_ton_hr * 1000 / 3600 # Convert tons/hr to kg/s
return K_r * ((1/D_final) - (1/D_initial)) * mass_flow_rate_kg_s
# Example parameters
D_initial_mm = 10 # Initial diameter of the feed material (in millimeters)
D_final_mm = 5 # Final diameter after crushing (in millimeters)
mass_flow_rate_ton_hr = 100 # Mass flow rate of the material (in tons per hour)
# Different values of Rittinger's constant (in kW*hr*m/ton)
K_r_values_kW_hr_m_ton = [0.5, 1.0, 1.5, 2.0, 2.5]
# Calculate power consumption for each K_r value
power_consumptions_kW = []
for K_r in K_r_values_kW_hr_m_ton:
power_consumption_kW = calculate_power_consumption(K_r, D_final_mm, D_initial_mm, mass_flow_rate_ton_hr)
power_consumptions_kW.append(power_consumption_kW)
# Plotting
plt.plot(K_r_values_kW_hr_m_ton, power_consumptions_kW, marker='o')
plt.xlabel("Rittinger's Constant (K_r) (kW*hr*m/ton)")
plt.ylabel("Power Consumption (kW)")
plt.title("Effect of Rittinger's Constant on Power Consumption")
plt.grid(True)
plt.show()
Output:
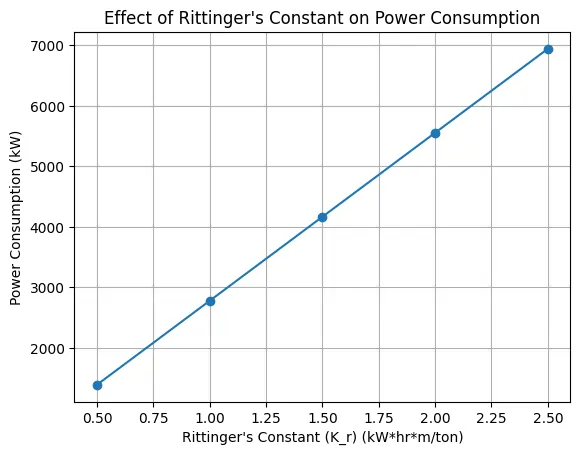
Resources:
- “Unit Operations in Chemical Engineering” (McCabe et al.)
- “Particle Technology and Separation Processes” (Richardson et al.)
- “Chemical Engineering Design: Principles, Practice and Economics of Plant and Process Design” (Towler and Sinnott)
Disclaimer: The Solver provided here is for educational purposes. While efforts ensure accuracy, results may not always reflect real-world scenarios. Verify results with other sources and consult professionals for critical applications. Contact us for any suggestions or corrections.